Linked List
Linked List Definition
A linked list is a linear data structure, in which the elements are not stored at contiguous memory locations. Pointers are used to link elements. Like arrays, Linked List is a linear data structure. Unlike arrays, linked list elements are not stored at a contiguous location. Linked List also has some advantages over arrays such as dynamic size and easier insertion or deletion. Linked list can be visualized as a chain of nodes, where every node points to the next node.
These are the basic operations supported by a list.
These are the basic operations supported by a list.
- Insertion − Adds an element at the beginning of the list
- Deletion − Deletes an element at the beginning of the list
- Display − Displays the complete list
- Search − Searches an element using the given key
- Delete − Deletes an element using the given key
Types of Linked List
- Simple Linked List − Item navigation is forward only
- Doubly Linked List − Items can be navigated forward and backward
- Circular Linked List − Last item contains link of the first element as next and the first element has a link to the last element as previous
Double Linked List
Doubly Linked List is a variation of Linked list in which navigation is possible in both ways, either forward and backward easily.
Following are the basic operations supported by a list.
- Insertion − Adds an element at the beginning of the list
- Deletion − Deletes an element at the beginning of the list
- Insert Last − Adds an element at the end of the list
- Delete Last − Deletes an element from the end of the list
- Insert After − Adds an element after an item of the list
- Delete − Deletes an element from the list using the key
- Display forward − Displays the complete list in a forward manner
- Display backward − Displays the complete list in a backward manner
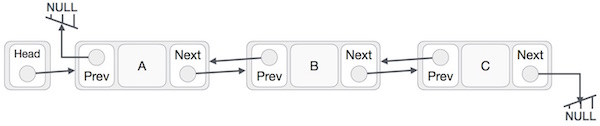
Circular Linked List
Circular Linked List is a variation of Linked list in which the first element points to the last element and the last element points to the first element. Both Singly Linked List and Doubly Linked List can be made into a circular linked list.
Following are the important operations supported by a circular list.
- insert − Inserts an element at the start of the list
- delete − Deletes an element from the start of the list
- display − Displays the list
Singly Linked List as Circular
In singly linked list, the next pointer of the last node points to the first node.

Doubly Linked List as Circular
In doubly linked list, the next pointer of the last node points to the first node and the previous pointer of the first node points to the last node making the circular in both directions.

Reference :
Comments
Post a Comment